初めてのArduino ④ UNOボードに表示器をつなぐ(その3 電圧を表示)
Arduino UNOにはアナログ入力があります。0~5Vを0~1023にA-D変換します。関数は、
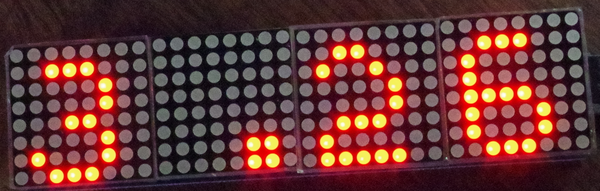
●スケッチ全部
#include <LEDMatrixDriver.hpp>
const uint8_t LEDMATRIX_CS_PIN = 10;
const int LEDMATRIX_SEGMENTS = 4;
const int LEDMATRIX_WIDTH = LEDMATRIX_SEGMENTS * 8;
int sensorPin = A0;
float Vcc = 4.38;
LEDMatrixDriver lmd(LEDMATRIX_SEGMENTS, LEDMATRIX_CS_PIN);
void setup() {
// init the display
lmd.setEnabled(true);
lmd.setIntensity(2); // 0 = low, 10 = high
}
// This is the font definition. You can use http://gurgleapps.com/tools/matrix to create your own font or sprites.
// If you like the font feel free to use it. I created it myself and donate it to the public domain.
byte font[95][8] = { {0,0,0,0,0,0,0,0}, // SPACE
{0x10,0x18,0x18,0x18,0x18,0x00,0x18,0x18}, // EXCL
{0x28,0x28,0x08,0x00,0x00,0x00,0x00,0x00}, // QUOT
{0x00,0x0a,0x7f,0x14,0x28,0xfe,0x50,0x00}, // #
{0x10,0x38,0x54,0x70,0x1c,0x54,0x38,0x10}, // $
{0x00,0x60,0x66,0x08,0x10,0x66,0x06,0x00}, // %
{0,0,0,0,0,0,0,0}, // &
{0x00,0x10,0x18,0x18,0x08,0x00,0x00,0x00}, // '
{0x02,0x04,0x08,0x08,0x08,0x08,0x08,0x04}, // (
{0x40,0x20,0x10,0x10,0x10,0x10,0x10,0x20}, // )
{0x00,0x10,0x54,0x38,0x10,0x38,0x54,0x10}, // *
{0x00,0x08,0x08,0x08,0x7f,0x08,0x08,0x08}, // +
{0x00,0x00,0x00,0x00,0x00,0x18,0x18,0x08}, // COMMA
{0x00,0x00,0x00,0x00,0x7e,0x00,0x00,0x00}, // -
{0x00,0x00,0x00,0x00,0x00,0x00,0x06,0x06}, // DOT
{0x00,0x04,0x04,0x08,0x10,0x20,0x40,0x40}, // /
{0x00,0x38,0x44,0x4c,0x54,0x64,0x44,0x38}, // 0
{0x04,0x0c,0x14,0x24,0x04,0x04,0x04,0x04}, // 1
{0x00,0x30,0x48,0x04,0x04,0x38,0x40,0x7c}, // 2
{0x00,0x38,0x04,0x04,0x18,0x04,0x44,0x38}, // 3
{0x00,0x04,0x0c,0x14,0x24,0x7e,0x04,0x04}, // 4
{0x00,0x7c,0x40,0x40,0x78,0x04,0x04,0x38}, // 5
{0x00,0x38,0x40,0x40,0x78,0x44,0x44,0x38}, // 6
{0x00,0x7c,0x04,0x04,0x08,0x08,0x10,0x10}, // 7
{0x00,0x3c,0x44,0x44,0x38,0x44,0x44,0x78}, // 8
{0x00,0x38,0x44,0x44,0x3c,0x04,0x04,0x78}, // 9
{0x00,0x18,0x18,0x00,0x00,0x18,0x18,0x00}, // :
{0x00,0x18,0x18,0x00,0x00,0x18,0x18,0x08}, // ;
{0x00,0x10,0x20,0x40,0x80,0x40,0x20,0x10}, // <
{0x00,0x00,0x7e,0x00,0x00,0xfc,0x00,0x00}, // =
{0x00,0x08,0x04,0x02,0x01,0x02,0x04,0x08}, // >
{0x00,0x38,0x44,0x04,0x08,0x10,0x00,0x10}, // ?
{0x00,0x30,0x48,0xba,0xba,0x84,0x78,0x00}, // @
{0x00,0x1c,0x22,0x42,0x42,0x7e,0x42,0x42}, // A
{0x00,0x78,0x44,0x44,0x78,0x44,0x44,0x7c}, // B
{0x60,0x60,0x1c,0x12,0x20,0x20,0x12,0x0c}, // C
{0x00,0x7c,0x42,0x42,0x42,0x42,0x44,0x78}, // D
{0x00,0x78,0x40,0x40,0x70,0x40,0x40,0x7c}, // E
{0x00,0x7c,0x40,0x40,0x78,0x40,0x40,0x40}, // F
{0x00,0x3c,0x40,0x40,0x5c,0x44,0x44,0x78}, // G
{0x00,0x42,0x42,0x42,0x7e,0x42,0x42,0x42}, // H
{0x00,0x7c,0x10,0x10,0x10,0x10,0x10,0x7e}, // I
{0x00,0x7e,0x02,0x02,0x02,0x02,0x04,0x38}, // J
{0x00,0x44,0x48,0x50,0x60,0x50,0x48,0x44}, // K
{0x00,0x40,0x40,0x40,0x40,0x40,0x40,0x7c}, // L
{0x00,0x82,0xc6,0xaa,0x92,0x82,0x82,0x82}, // M
{0x00,0x42,0x42,0x62,0x52,0x4a,0x46,0x42}, // N
{0x00,0x3c,0x42,0x42,0x42,0x42,0x44,0x38}, // O
{0x00,0x78,0x44,0x44,0x48,0x70,0x40,0x40}, // P
{0x00,0x3c,0x42,0x42,0x52,0x4a,0x44,0x3a}, // Q
{0x00,0x78,0x44,0x44,0x78,0x50,0x48,0x44}, // R
{0x00,0x38,0x40,0x40,0x38,0x04,0x04,0x78}, // S
{0x00,0x7e,0x90,0x10,0x10,0x10,0x10,0x10}, // T
{0x00,0x42,0x42,0x42,0x42,0x42,0x42,0x3e}, // U
{0x00,0x42,0x42,0x42,0x42,0x44,0x28,0x10}, // V
{0x80,0x82,0x82,0x92,0x92,0x92,0x94,0x78}, // W
{0x00,0x42,0x42,0x24,0x18,0x24,0x42,0x42}, // X
{0x00,0x44,0x44,0x28,0x10,0x10,0x10,0x10}, // Y
{0x00,0x7c,0x04,0x08,0x7c,0x20,0x40,0xfe}, // Z
// (the font does not contain any lower case letters. you can add your own.)
}; // {}, //
int sensorValue;
void loop(){
sensorValue = analogRead(sensorPin);
float analogSensorData = Vcc * sensorValue / 1024.0;
String sensorValueSTR = String(analogSensorData, DEC);
char Buf[10];
sensorValueSTR.toCharArray(Buf, 10);
drawString(Buf, 4, 0, 0);
// Toggle display of the new framebuffer
lmd.display();
delay(2000);
}
/**
* This function draws a string of the given length to the given position.
*/
void drawString(char* text, int len, int x, int y )
{
for( int idx = 0; idx < len; idx ++ )
{
int c = text[idx] - 32;
// stop if char is outside visible area
if( x + idx * 8 > LEDMATRIX_WIDTH )
return;
// only draw if char is visible
if( 8 + x + idx * 8 > 0 )
drawSprite( font[c], x + idx * 8, y, 8, 8 );
}
}
/**
* This draws a sprite to the given position using the width and height supplied (usually 8x8)
*/
void drawSprite( byte* sprite, int x, int y, int width, int height )
{
// The mask is used to get the column bit from the sprite row
byte mask = B10000000;
for( int iy = 0; iy < height; iy++ )
{
for( int ix = 0; ix < width; ix++ )
{
lmd.setPixel(x + ix, y + iy, (bool)(sprite[iy] & mask ));
// shift the mask by one pixel to the right
mask = mask >> 1;
}
// reset column mask
mask = B10000000;
}
}
●見やすくする
エディット領域の右にある三角をクリックして、New Tabを選びます。
名前を付けます。fontsとしました。
fonts.inoというタブができるので、fontテーブル部分とloop()の下側にあったvoid drawString()、void drawSprite()をカットして持っていきます。
メイン部分はsetup()、loop()だけになり、すっきりしました。プログラムを分けた部分の読み込むためのincludeとかの記述は不要です。このままコンパイルできます。
●型
スケッチの最初のほうで、いろいろな型の指定が混在しています。きれいではありません。昔の書き方で統一しました。
intはArduino UNOとMKR Zeroでは長さが異なるかもしれません。例えば16ビットと32ビット。そういう不安がある場合は、きっちりと指定できます。intの型の中でint8_tは1バイトで、-128 ~ 127の範囲の数値が扱えます。int16_tは2バイトで、-32,768 ~ 32,767の範囲の数値が扱えます。
上記より、フラッシュ・メモリ=ROMは16バイト小さくなりました。RAMも1バイト余裕ができました。
これ以外に、プログラムのスピードがそのCPUで速くなるバイト数が確保されるint_fastX_t、格納可能な最も小さいサイズが確保されるint_leastX_tがありますが、X=8では1バイトになるようです(未確認)。Arduinoのスケッチで使っているのを見たことはありません。
定数などでは文字の置き換えを利用できます。#defineを使ったからといって、それほどメモリの消費は変わらないようです。